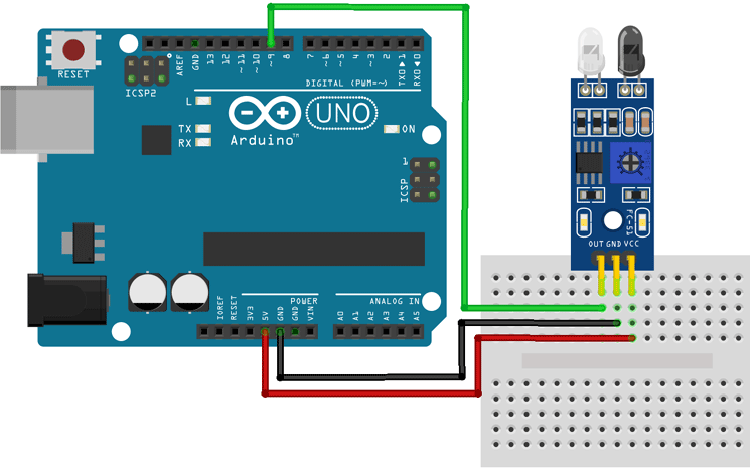
What is an IR Sensor?
An Infrared (IR) sensor is an electronic device that detects infrared radiation emitted by objects in its field of view. It typically consists of an IR LED (transmitter) and a photodiode (receiver). The IR LED emits infrared light, which is invisible to the human eye. When an object passes in front of the sensor, the emitted light reflects off the object and is detected by the photodiode.
IR sensors are commonly used for proximity detection, obstacle detection, and motion sensing. They are widely found in remote control systems, automatic doors, line-following robots, and various home automation systems.
To interface an Infrared (IR) sensor with an Arduino, you'll need the following components:
Components:
Arduino (any model, like Uno)
IR Sensor module
Jumper wires
Breadboard (optional)
Wiring the IR Sensor to the Arduino:
An IR sensor usually has 3 pins: VCC, GND, and OUT (or DATA). Here’s how you can connect them:
VCC → 5V pin on Arduino
GND → GND pin on Arduino
OUT (Signal Pin) → Digital Pin (e.g., D9)
Code Example:
int sensorPin = 9; // Pin where the IR sensor is connected
int sensorValue = 0; // Variable to store the sensor reading
void setup() {
pinMode(sensorPin, INPUT); // Define the sensor pin as input
Serial.begin(9600); // Initialize serial communication for debugging
}
void loop() {
sensorValue = digitalRead(sensorPin); // Read the value from the sensor (HIGH/LOW)
if (sensorValue == HIGH) { // If IR detects an object
Serial.println("Object Detected");
} else { // If no object is detected
Serial.println("No Object");
}
delay(100); // Small delay for stability
}
How the Code Works:
pinMode(sensorPin, INPUT): Sets the IR sensor pin as an input pin.
digitalRead(sensorPin): Reads the signal from the IR sensor (either HIGH or LOW).
If the sensor detects an object (usually reflecting IR rays), it outputs HIGH; otherwise, it outputs LOW
Testing:
After wiring, upload the code to your Arduino using the Arduino IDE.
Open the Serial Monitor (Ctrl + Shift + M) to observe the "Object Detected" or "No Object" messages as you place objects in front of the IR sensor.
Comments